Password Generator in Python
- Kwnstantinos Lambrou
- Jan 21
- 2 min read
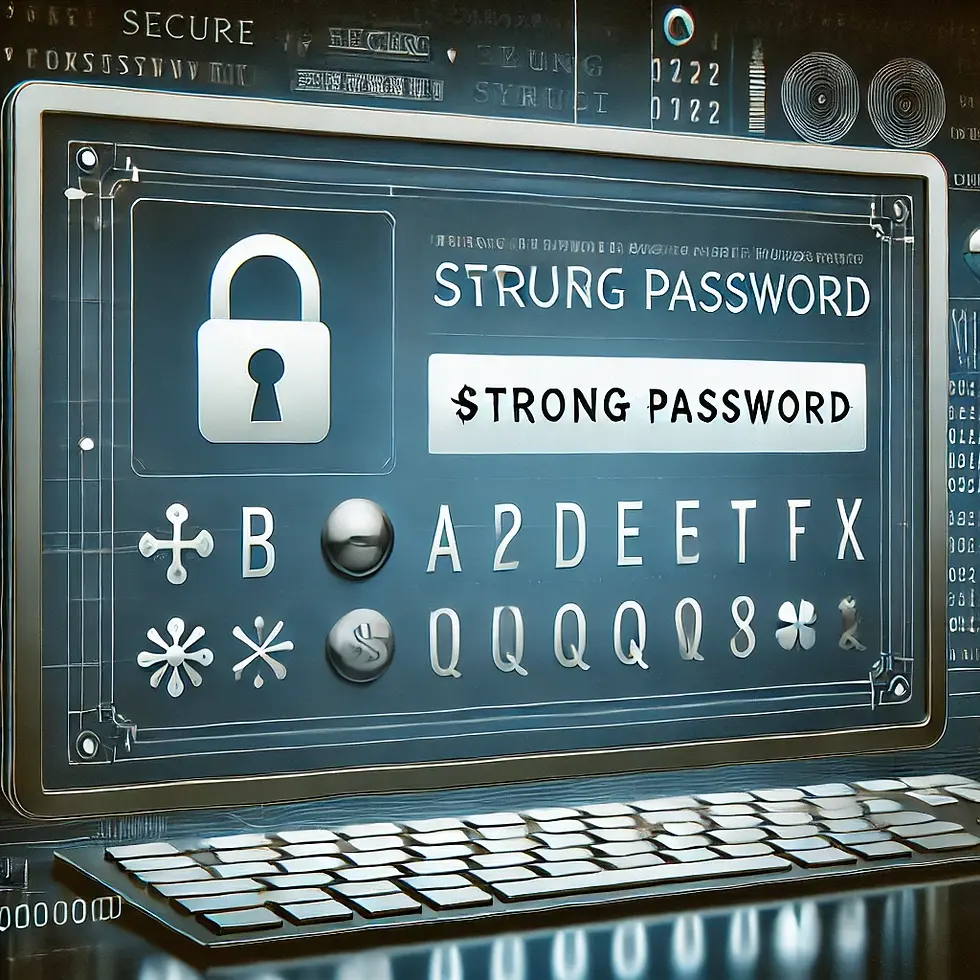
Welcome to my mini Python project, where I have developed an automated password generator. This utility creates secure, random passwords based on user-defined criteria.
Project Description
The password generator is designed to create a secure password that meets specific requirements. Here’s how the code works:
Function Definition: The function generate_password is defined to accept three parameters:
min_length: The minimum length of the password.
numbers: A boolean indicating whether to include numeric characters in the password.
special_characters: A boolean indicating whether to include special characters like punctuation.
Character Set Initialization:
letters = string.ascii_letters: Includes all alphabet characters (both uppercase and lowercase).
digits = string.digits: Includes numeric characters from 0 to 9.
special = string.punctuation: Includes special characters such as !@#$%^&*(), etc.
Character Pool Creation:
The character pool starts with just letters.
If numbers is True, digits are added to the pool.
If special_characters is True, special characters are added.
Password Generation Loop:
A password string pwd is initialized as empty.
The loop continues until the generated password meets the criteria of having numbers and/or special characters if required, and until it reaches the minimum length.
random.choice(characters) selects a random character from the combined pool and adds it to the password string.
Flags has_number and has_special check if the password contains at least one number or special character, respectively.
User Input:
The user is prompted to enter the desired minimum length and whether the password should include numbers and special characters.
Output:
The final password is printed out, showing a secure string that matches the user’s requirements.
View the Code
To see the full code for this project and possibly contribute or fork the repository for your use, please visit my GitHub repository.
Comments